dScope Series III Scripting and automation
Scripting Basics: anatomy of a dScope script
This page is intended as a basic introduction to scripting with VBScript, particularly within the dScope environment. The intention is not to replace the wealth of other material out there for VBScript, but to provide a little assistance in getting starting scripting dScope. For a practical introduction to scripting with dScope, see also the Scripting: Getting Started page.dScope .dss scripts
dScope scripts have the file extension .dss, and are essentially VBScripts with a few extra enhancements for use with dScope. All the programming is done in VBScript and the Microsoft VBScript reference material and information on the internet for VBScript should apply. Here we are going to take a look at the anatomy of a simple dScope script and take it apart line by line in order to better understand how it works.
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 |
' TYPE Automation ' LANGUAGE VBScript ' DESCRIPTION Example Script ' *** Declarations *** Option Explicit ' Must declare all variables before using them Dim dReading 'The Signal Analyzer RMS amplitude Reading from Channel A in dBu Dim strTitle 'The title string for message boxes etc. '*** Initialisation *** strTitle = "Script example" ' *** Main body of script *** Sub dScope_Main ' *** TODO : Insert your code here *** SignalGenerator.SG_ChAFreqUnit = UNIT_FREQ_HZ SignalGenerator.SG_ChAFreq = 200 dReading = SignalAnalyzer.SA_ChARMSAmpl MsgBox "Amplitude = " & Round(dReading,2) & "dBu", vbInformation, strTitle ' Remove next line if script should ' continue responding to events Automation.AUT_StopScript() End Sub ' dScope_Main |
Type Declaration: Line 001
This is a dScope specific line. As far as VBScript is concerned, this is simply a comment, but dScope reads this in order to determine the type of script it is. There are differences in execution depending on the type of script. This script is being declared as an Automation script. See the dScope "Scripting Manual" topic "Types of dScope Script" for more information on this.
Language Declaration: Line 002
Again this is a dScope specific line that, as far as VBScript is concerned, is simply a comment. dScope can be scripted in either VBScript or JScript. In practice, nearly all scripts will be VBScript and this line will be as shown.
Description comment: Line 003
This is simply a comment used to describe the script. Any text preceded by a single quote is a comment.
Option Explicit Statement: Line 006
This line tells the script engine that you require each variable that you are going to use to be declared explicitly. We'll discuss variables in the next step. This line has to be the first executable line in the script (ie, the first line that is not a comment). In the lines below it, we will be declaring our variables using the "Dim" statement. Using Option Explicit helps to avoid using mis-typed variables or variables whose scope is not clearly defined. (Where you declare the variable determines where it can be used and this is known as it's "scope")
Declaring Variables: Lines 007 and 008
A "variable" is a place-holder or "container" that refers to a computer memory location where you can store program information that may change during the time your script is running. Here we are declaring the variables using the "Dim" statement. "Dim" comes from the word "Dimension" and referred originally to setting up the required dimensions in memory for the type of data that were to be stored. In some programming languages you have to tell the program what type of data the variable will hold. In VBScript this is done automatically behind the scenes based on the kind of data you put in it so you don't have to declare a data type. Having said that, it's a good idea when declaring variables to add a comment saying what the variable is, and if appropriate, what units it's in. The variable names must begin with a letter, cannot contain full stops/periods (.) or spaces, must be unique and must be less than 255 characters long. Although they are not case sensitive, it is a good idea to keep the case consistent as it can help with search and replace operations later.
Initialising Variables: Line 011
Quite often you may want to use a variable throughout your script to save you having to re-type the same thing over and over again. As an example, here we are using the variable "strTitle" to hold the name that we want to appear at the top of message boxes etc. Doing it this way means that we can have hundreds of message boxes and they can all have the same title, or a variation on it, without having to re-type it each time. It also means we can change the name that appears on all the message boxes from the script by changing it in one place.
Many variables don't need to be initialised. We are not going to bother to initialise dReading as we are going to set its value in the script later.
The dScope_Main Subroutine: Lines 014 to 028
While it's not essential to have a dScope_Main subroutine in a dScope script and you could put commands outside this (for example, at line 012), the dScope_Main has some special significance for running dScope scripts, in particular when it comes to responding to dScope events. (An "event" in this context is a computer message that is generated behind the scenes when some event happens. Scripts can respond to events such as dScope limits being breached, but only under certain conditions - see the dScope scripting manual for more information.)
Subs are self-contained defined procedures and contain code that executes when they are "called". You would call a sub called "Get_New_Reading" by adding the line "Call Get_New_Reading". As the script is executed, when it hits this "Call" statement, it goes off and runs the sub referred to and then comes back and continues executing with the next line down from the call statement. If you repeatedly use the same short procedure throughout a script, it can be useful to put it in a sub and then call it when you need it. Subs can be called from within other subs. The naming conventions for subs are the same as for variables.
Setting a property: Lines 017 and 18
Line 017 is the first bit of this script that actually refers to the dScope object. In this context, an "object" is a programming entity that you can access to get it to do things (it's "methods") or change its settings (it's properties.) Within a .dss dScope script you don't need to create a link to the dScope object - it already knows about it, so you only have to tell the script which aspect of the dScope you are interested in controlling. Here the line "SignalGenerator.SG_ChAFreqUnit" says we are interested in changing the "SG_ChAFreqUnit" property of the "SignalGenerator" object and setting it to the value "UNIT_FREQ_HZ". If accessing this from outside the dScope, the Signal Generator would be a child of the dScope object and you would write it something like "dScope.SignalGenerator.SG_ChAFreqUnit" where the full stop/period indicates that the SignalGenerator belongs to the dScope object, and the "SG_ChAFreqUnit" is a property of the Signal Generator.
Line 017 also uses a dScope "constant" called "UNIT_FREQ_HZ". A "constant" is a like variable that is defined at some point within the dScope software. Here we can think of it as the name of the position of a switch that determines some option within the dScope. In this instance behind the scenes the constant called "UNIT_FREQ_HZ" actually is an integer with the value 11. Although we could say "SignalGenerator.SG_ChAFreqUnit = 11" and the result would be the same, this is not good practice as the definition of the constant could change within the dScope software with a new version. Referring to it by its constant name also allows us to more easily see what is happening as the name will tend to describe the setting we are changing.
Line 018 shows setting the value of the dScope Generator Frequency in Hz. Note that this line doesn't say anything about the units the generator frequency is to be set in. The value passed to this property is in whatever units the property is in at the time, so care must be taken to make sure we know what we are actually setting. Note also here that the value 200 is not in quotes. This is because it is a number and not a "string" (text). As a general rule, anything that you enclose in quotes is interpreted as being text, and anything that is not in quotes is a value. VBScript is pretty good at working out what you intend with strings and numerical values, but if you get an error message about "Type mismatch" this is quite likely to be the cause.
Reading a property: Line 020
This line is setting the value of the variable "dReading" to the value of the dScope Signal Analyzer Channel A RMS reading. Again, this variable has no defined units - it's whatever units the dScope Signal Analyzer is working in at the time. Here we have assumed that it is in dBu without specifically setting it which may be a safe assumption if we have recently loaded a dScope configuration that has it set in dBu. It's good practice to define what the units should be when the variable is declared so that it is straight-forward to check later that the script is working consistently with the dScope settings.
Displaying the result in a message box: Line 022
The message box function is a VBScript means of displaying a Windows style message box on screen. It has only three parameters which are separated by commas. The first is the text that is to be displayed, and this is the only required parameter. The other two are the message box type (here we are setting it to be an "information" message box), and the title, which we are setting to be the value of our variable "strTitle" which we defined earlier. An example of the result of running this script is shown below:
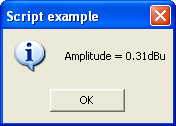
The "Round" function is used to round the value of dReading which we took earlier to 2 decimal places, and we are constructing a longer string to display as a message by combining different bits of text together using the "&" operator.
Stopping the Script: Line 026
In this instance the line "Automation.AUT_StopScript()" is not strictly necessary as the script will just complete and stop by itself. In some more complex scripts, this can be used to stop a script from executing.
Summary
Obviously this has had to be a fairly basic look at dScope scripting and has barely scratched the surface. We haven't discussed flow control, functions, input boxes, user interfaces etc. etc., but it has shown the fundamentals for getting a script to work. From here, it's a matter of building knowledge of the VBScript language in order to construct more useful scripts, and a deeper understanding of the dScope software to be able to dig deeper into what is available to control and measure. Beyond that, there is accessing other programs, accessing the Registry, manipulating files, generating results as web pages etc. etc.
Further Reading
- Scripting: Getting started - a very basic step by step introduction
- dScope Scripting Page - scripting examples and resources including links to the Microsoft VBScript documentation
- Download the Scripting manual - the full dScope scripting and automation reference
Microsoft Resources
Links to various technical resources on the MSDN (Microsoft Developers Network) website - these links open in a new window or tab.
- On-line guide - MS script technologues
- Scripting Runtime documentation
- Script components
- The Windows Scripting Host
- Windows Script Interfaces
- VBScript - as embedded in dScope