dScope Series III Scripting and automation
Creating Custom Controls using ScriptDlg.DLL
OverviewAlthough VBScript is very powerful for many aspects of automation of dScope, it natively lacks any means of creating user controls. There are many instances when we would like to be able to control the settings of script, or view information in a different form. In order to be able to do this from a script, the dScope installation includes a special DLL (Dynamic Link Library) which provides the capability of creating custom controls from a dScope script.
The basics
A ScriptDlg object is created from a script using the standard methods for creating ActiveX controls - using "CreateObject". This function will return a reference to the object in question, which is then used in every further operation involving the ScriptDlg object.
Set form = CreateObject("ScriptDlg.Form") |
Once the form object has been created, you will need to decide whether you want to handle events in your form (button clicks, list box selections, etc). This will usually be the case, so you will need to initialise the object's Event Handler. To do this, pass the object you just created to the InitEventHandler method:
form.InitEventHandler(form) |
Now it's time to add some controls. To do this, you need to call Add... for each control that you want in your dialogue box:
form.AddStatic "static1", "This is some text" form.AddPushButton "button1", "Click me" |
Note that the first parameter to each of these Add... methods is the name of the button. This will be the name used when referring to the control throughout the script.
Finally, now our controls are set up, we need to tell the ActiveX control to actually display the dialogue box:
form.Display() |
Note that you don't have to tell the dialogue where to put the controls, as it positions and sizes them automatically. It is possible to set where each control is positioned but if no position information is added, the script defaults to adding them next to each other. The whole script to do this if done in the dScope editor would look like this:
' TYPE Automation ' LANGUAGE VBScript ' DESCRIPTION ' *** Declarations *** Option Explicit ' Must declare all variables before using them Dim Form ' *** Main body of script *** Sub dScope_Main ' *** TODO : Insert your code here *** Set form = CreateObject("ScriptDlg.Form") form.InitEventHandler(form) form.AddStatic "static1", "This is some text" form.AddPushButton "button1", "Click me" form.Display() ' Remove next line if script should ' continue responding to events Automation.AUT_StopScript() End Sub ' dScope_Main ' *** Event handlers *** |
If you run the script, you should now see a dialogue box that looks something like the following:
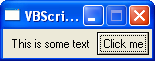
While this looks nice, it's not actually very useful as the button doesn't do anything. To make the button do something, we need to tell the script what sub to call when it is clicked, and then define that sub. To do this we add the following line: (it goes just below the line in the script where the button has been defined)
form.button1.SetEvent_OnClick GetRef("button1_OnClick") |
And then, at the end of the script in the "event handlers" section:
Sub button1_OnClick() MsgBox "You clicked the button!", vbinformation End Sub |
When this is done, when the button is clicked, the sub button1_OnClick() will execute and a message box will appear saying "You clicked the button!" - still not very exciting, but now the possibilities are open to do almost anything: the sub could contain dScope scripting to run a test, change dScope settings etc.
The Form
The tables below summarize the available properties and methods of the ScriptDlg form, and the available controls. Note that this is a guide to the available features rather than official documentation, for this reason the "options" listed in the controls section are not necessarily the actual names of the methods or properties, but an indication of what can be done. For details of how to use the methods and properties of the controls, please see the dScope Scripting Manual.
|
ScriptDlg Controls
Below is a quick summary of the available controls and what they can do:
Note that this is a guide to the available features rather than official documentation, for this reason the "options" listed are not necessarily the actual names of the methods or properties, but an indication of what can be done. For details of how to use the methods and properties of the controls, please see the dScope Scripting Manual.
Getting Help
The SciptDlg.dll documentation is included in the dScope help in the dScope Scripting Manual, in the "ScriptDlg ActiveX control" section. More general VBScript help is available from the Microsoft web site. The ScriptDlg methods and properties are not listed with the rest of the dScope methods and properties in the dScope edit window although they can be made available in some editors if you add ScriptDlg.DLL to the list of references. Note that there are numerous examples of ScriptDlg controls in the dScope installation as they are used for most of the applications such as AES17, Dolby and DTS tests. Any of these can be opened in the editor and the code copied or adapted. You can also email Prism Sound technical support for more assistance.